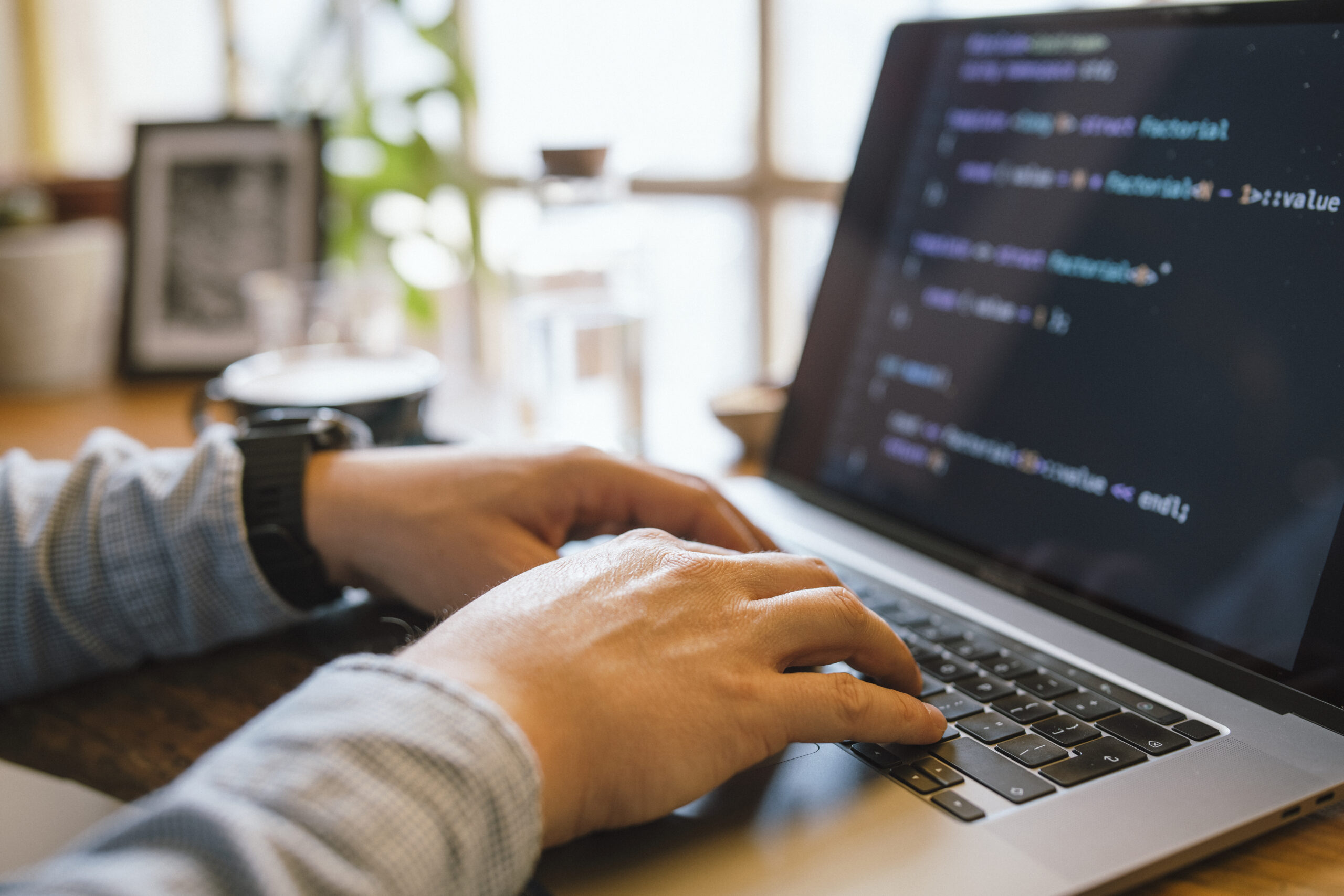
Debugging is one of the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why issues go Improper, and Finding out to Consider methodically to resolve challenges successfully. Irrespective of whether you are a starter or a seasoned developer, sharpening your debugging abilities can save hours of disappointment and drastically boost your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is one Element of progress, being aware of the best way to interact with it successfully during execution is Similarly crucial. Contemporary enhancement environments appear equipped with powerful debugging abilities — but several developers only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When applied correctly, they Enable you to observe precisely how your code behaves during execution, and that is priceless for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep an eye on network requests, look at actual-time general performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change disheartening UI concerns into workable tasks.
For backend or technique-level builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command in excess of running processes and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Manage programs like Git to be familiar with code background, uncover the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The greater you realize your resources, the more time you'll be able to devote fixing the actual dilemma as an alternative to fumbling by the procedure.
Reproduce the situation
Among the most essential — and sometimes neglected — methods in powerful debugging is reproducing the challenge. In advance of jumping in to the code or creating guesses, developers have to have to produce a regular surroundings or situation where the bug reliably seems. With no reproducibility, repairing a bug gets to be a game of prospect, generally resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating just as much context as is possible. Check with inquiries like: What actions triggered The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you may have, the less difficult it becomes to isolate the precise situations under which the bug happens.
When you’ve gathered adequate details, try to recreate the situation in your local ecosystem. This might necessarily mean inputting the exact same information, simulating identical consumer interactions, or mimicking procedure states. If the issue seems intermittently, look at creating automatic checks that replicate the edge scenarios or state transitions concerned. These checks not just support expose the problem but in addition reduce regressions Later on.
From time to time, the issue can be atmosphere-distinct — it'd occur only on specified operating methods, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating such bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It demands persistence, observation, plus a methodical solution. But once you can regularly recreate the bug, you are previously midway to correcting it. That has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse additional Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete problem — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes wrong. Rather than looking at them as disheartening interruptions, builders need to find out to treat error messages as direct communications within the procedure. They generally inform you just what occurred, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept very carefully and in whole. A lot of developers, specially when beneath time stress, look at the primary line and instantly get started building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like yahoo — browse and recognize them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it point to a particular file and line selection? What module or operate triggered it? These issues can guidebook your investigation and issue you toward the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context in which the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These frequently precede much larger concerns and supply hints about opportunity bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, aiding you pinpoint difficulties faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful resources inside a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
A superb logging approach commences with recognizing what to log and at what amount. Prevalent logging concentrations involve DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for standard activities (like productive begin-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Deal with essential activities, state changes, enter/output values, and demanding decision factors within your code.
Structure your log messages Obviously and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. Which has a effectively-assumed-out logging strategy, you could reduce the time it requires to identify issues, achieve further visibility into your applications, and improve the Over-all maintainability and reliability of one's code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To successfully discover and take care of bugs, builders should technique the method similar to a detective resolving a mystery. This attitude will help stop working complicated concerns into manageable pieces and follow clues logically to uncover the root result in.
Commence by collecting proof. Think about the symptoms of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent data as it is possible to devoid of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a transparent photo of what’s taking place.
Up coming, kind hypotheses. Request oneself: What could possibly be leading to this behavior? Have any changes recently been here made into the codebase? Has this difficulty transpired ahead of beneath equivalent situations? The goal is to slender down opportunities and recognize likely culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a controlled ecosystem. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out close consideration to little aspects. Bugs typically conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the actual trouble, just for it to resurface later.
Last of all, hold notes on what you experimented with and discovered. Just as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical competencies, method troubles methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Generate Tests
Creating assessments is among the simplest approaches to help your debugging skills and All round progress performance. Checks not only assist catch bugs early but in addition function a security net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Performing as predicted. Every time a examination fails, you quickly know the place to seem, drastically lowering time invested debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear following previously staying fastened.
Up coming, integrate integration checks and close-to-conclude exams into your workflow. These help make sure several areas of your application work jointly easily. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline failed and beneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to deal with fixing the bug and enjoy your exam pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable process—assisting you catch far more bugs, faster and much more reliably.
Get Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—observing your monitor for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just hours before. During this point out, your Mind gets fewer economical at trouble-resolving. A short walk, a coffee crack, as well as switching to a distinct activity for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also support stop burnout, especially all through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that point to maneuver all around, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, but it in fact results in a lot quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a wise system. It provides your Mind space to breathe, improves your point of view, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to develop being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can teach you one thing precious if you take some time to mirror and examine what went Erroneous.
Get started by asking your self several essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or comprehension and make it easier to Make more robust coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or frequent blunders—that you could proactively steer clear of.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, a lot of the ideal builders will not be those who publish perfect code, but individuals who continuously understand from their errors.
In the long run, Every bug you deal with adds a whole new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.